E-mail Me
Syllabus
Schedule
Handouts
Assignments
All My Webs
Dept Home

|
Programming Assignment 1
Purpose: This assignment is intended to demonstrate your skills with
:
- Variable declaration and assignment
- InputBox and MsgBox functions,
- text manipulation and concatenation
- the If-Then-Else decision structure
The completed assignment should do the following:
-
Using InputBox, ask the
user for their first name, last name, and Dragon ID number.
-
Store the input values
in separate memory variables named vFirst, vLast, and vID, respectively.
-
Use a message box to ask
the user if the displayed values are correct. If they respond
"Yes", move on to step 4. If not, have the macro display
a message and stop.
-
Determine a user code
by joining the first three letters of the users last name, and
the first letter of the users first name with the last 4 digits
of their Dragon ID number. For example, if John Smiths Dragon
ID is 00124578, his code should be "SMIJ4578".
-
Use a message box to display
the users name (Last name, First name) and their user code.
This project is worth 20 points. The grading for
this project will be as follows:
To earn 70 percent (14 points) your program must::
- Use the DIM declaration to create five text variables, vFirst, vLast,
vid, vOut, and vCode
- Pop up three input boxes to ask for the user's first
name, last name, and Dragon ID
- Use assignment statements to store the collected information in the
appropriate variable.
- Use MsgBox to display the data as the user entered
it:
- Use text strings and the ampersand (&) to display each item
with a label
i.e. First Name: Ron
- Use the vbCrLf constant to display each piece of
information on its own line.
- Store the completed text string in the variable vOut
- Use MsgBox to display the message in vOut
Your message box may look like this:
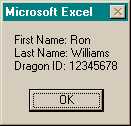
To earn 80% (16 points), do the above plus:
- Using the Right( ) and Left( ) functions, get the macro
to generate the user code.
- Use an assignment statement to store the user code
in vCode
To earn 90% (18 points), do the above plus:
- Create a text string to display the user's name, last name first (Smith,
John).
- Use MsgBox to display the user's full name and user
code.
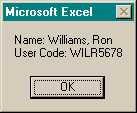
To earn 100% (20 points), do the above plus:
- Expand the MsgBox function in the 70% assignment to display two buttons
marked Yes and No.
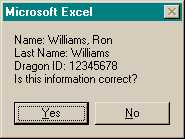
- Using an If-Then-Else statement, ask the user
if their data is acceptable.
- If they answer yes, display the MsgBox described
in the 90% assignment above.
- If not, display a message asking the user to start
again, and stop the macro.
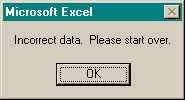
|